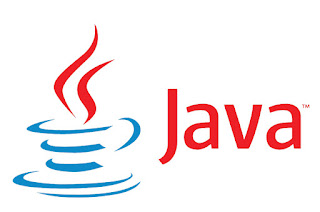
1. What is the use of JSESSIONID in web application?
JSESSIONID cookie is created/sent when session is created. Session is created when your code calls request.getSession()
or request.getSession(true)
for the first time. If you just want to get the session, but not create it if it doesn't exist, use request.getSession(false)
this will return you a session or null. In this case, new session is not created, and JSESSIONID cookie is not sent. (This also means that session isn't necessarily created on first request... you and your code are in control when the session is created)
In Java J2EE application container is responsible for Session management and by default uses Cookie. When a user first time access your web application, session is created based on whether its accessing HTML, JSP or Servlet. if user request is served by Servlet than session is created by calling request.getSession(true) method. it accepts a boolean parameter which instruct to create session if its not already existed.
If you call request.getSession(false)
then it will either return null if no session is associated with this user or return the associated HttpSession object. If HttpRequest is for JSP page than Container automatically creates a new Session with JSESSIONID if this feature is not disabled explicitly by using page directive <%@ page session="false" %>
.
You can check the value of JSESSIONID coming in as cookie by monitoring HTTP request. If you are running Tomcat Server in NetBeans IDE in your development environment then you can use HTTP Server Monitor to check HTTP requests. You just need to enable it while starting Tomcat Server form Netbeans. After than with each request you can see all details of request headers, session, cookies etc in HTTP Server monitor screen. If you look on JSESSIONID cookie it will look like:
cookie JSESSIONID=1A530637289A03B07199A44E8D531427
2. What is load-on-startup servlet element in web.xml?
load-on-startup is a tag element which appear inside <servlet>
tag in web.xml. load-on-startup tells the web container about loading of a particular servlet. if you don't specify load-on-startup then container will load a particular servlet when it feels necessary most likely when first request for that servlet will come, this may lead to longer response time for that query if Servlet is making database connections or performing ldap authentication which contribute network latency or any other time consuming job, to avoid this, web container provides you a mean to specify certain servlet to be loaded during deployment time of application by using load-on-startup parameter.
If you specify load-on-startup parameter inside a servlet than based upon its value Container will load it.you can specify any value to this element but in case of load-on-startup>0 , servlet with less number will be loaded first. For example in below web.xml AuthenticationServlet will be loaded before AuthorizationServlet because load-on-startup value for AuthenticationServlet
is less (2) while for AuthorizationServlet is 4.
Important points on load-on-startup element
- If
<load-on-startup>
value is same for two servlet than they will be loaded in an order on which they are declared inside web.xml file. - if
<load-on-startup>
is 0 or negative integer than Servlet will be loaded when Container feels to load them.<load-on-startup>
guarantees loading, initialization and call to init() method of servlet by web container. - If there is no
<load-on-startup>
element for any servlet than they will be loaded when web container decides to load them.
3. What is JSTL forTokens Tag?
JSTL forTokens tag is another tag in core JSTL library to support Iteration or looping. It effectively complements, more useful <c:forEach>
tag, by allowing you to iterate over comma separated or any delimited String. You can use this tag to split string in JSP and can operate on them individually.
Here is our complete code example of using JSTL forTokens tag in JSP page. In this example, we first split a comma separate String using forTokens tag by specifying delims=";". When we iterate over tokens, var represent current token. In the second example, we have specified multiple delimiter in delims attribute, delims="|," to split String by pipe(|) character and comma (,) character.
4. Difference between SendRedirect() and Forward()?
SendRedirect ():
This method is declared in HttpServletResponse Interface.
Signature: void sendRedirect(String url)
difference between sendRedirect and forward in jsp servletThis method is used to redirect client request to some other location for further processing ,the new location is available on different server or different context.our web container handle this and transfer the request using browser ,and this request is visible in browser as a new request. Some time this is also called as client side redirect.
Forward():
This method is declared in RequestDispatcher Interface.
Signature: forward(ServletRequest request, ServletResponse response)
This method is used to pass the request to another resource for further processing within the same server, another resource could be any servlet, jsp page any kind of file.This process is taken care by web container when we call forward method request is sent to another resource without the client being informed, which resource will handle the request it has been mention on requestDispatcher object which we can get by two ways either using ServletContext or Request. This is also called server side redirect.
RequestDispatcher rd = request.getRequestDispatcher("pathToResource"); rd.forward(request, response);Or
RequestDispatcher rd = servletContext.getRequestDispatcher("/pathToResource"); rd.forward(request, response);
5. How to Configure HTTPS (SSL) in Tomcat 6 and 7 Java Web Server?
As I explained you need to have some certificate (inside keystore) in tomcat/conf folder which tomcat will present, when a connection is made via https. If you use Spring security you can use some of test certificates present in there sample applications otherwise you need to generate by yourselves. You can request certificates from your windows support team or by using tools like IBM IkeyMan and keytool command to put them into truststore and keystore.
Once you have certificate ready, Open your server.xml from tomcat/conf folder and search for Connector which defines https, it may be commented ,better look for this string "Define a SSL HTTP/1.1 Connector on port 8443". Once found replace with following setup which is different for tomcat 6 and tomcat 7
SSL Configuration for Tomcat 6 :
<Connector protocol="org.apache.coyote.http11.Http11Protocol" port="8443" minSpareThreads="5" maxSpareThreads="75" enableLookups="true" disableUploadTimout="true" acceptCount="100" maxThreads="200" scheme="https" secure="true" SSLEnabled="true" clientAuth="false" sslProtocol="TLS" keystoreFile="${catalina.home}/conf/server.jks" keystoreType="JKS" keystorePass="changeit" />
You also need to make one more configuration change for setting up SSLEngine="off" from "on" like in below text:
<Listener className="org.apache.catalina.core.AprLifecycleListener" SSLEngine="off" />
Look for this String on top of Server.xml
SSL Configuration for Tomcat 7
SSL Setup in Tomcat7 is relatively easy as compared to Tomcat7, as you only need to make one configuration change for replacing SSL Connector with following settings :
<Connector port="8443" protocol="HTTP/1.1" SSLEnabled="true" maxThreads="150" scheme="https" secure="true" clientAuth="false" sslProtocol="TLS" keystoreFile="${catalina.home}/conf/server.jks" keystoreType="JKS" keystorePass="changeit" />Settings which may vary if you setup your own certificate is keystorFile which points to a keystore, which stores certificates, keyStoreType I am using "jks", which stands for “Java Key Store” and keystorepass, which is password for opening key store file. That's it now your tomcat 6 or tomcat 7 is ready to server https client. Though you may need to configure https for your web application ,if you not done already.
6. How to configure Java web application for https?
If you want your J2EE web application to be accessed over SSL using https protocol, you can include following settings in application's web.xml :
This Security setting will enable HTTPS for all URL directed your application. you can also selective enable https settings for some URL by tweaking URL pattern. Since SSL requires encryption and decryption it can increase response time and if you not serving sensitive information than you only have SSL enable for login or any particular URL which requires sensitive data.
7. Difference between Application Server and Web Server in Java?
- Application Server supports distributed transaction and EJB. While Web Server only supports Servlets and JSP. Application Server can contain web server in them. most of App server e.g. JBoss or WAS has Servlet and JSP container.
- Though its not limited to Application Server but they used to provide services like Connection pooling, Transaction management, messaging, clustering, load balancing and persistence. Now Apache tomcat also provides connection pooling.
- In terms of logical difference between web server and application server. web server is supposed to provide http protocol level service while application server provides support to web service and expose business level service e.g. EJB.
- Application server are more heavy than web server in terms of resource utilization.
8. Difference between Servlet and JSP?
- The first and foremost difference between Servlet and JSP is that a JSP is a web page scripting language that can generate dynamic content while Servlets are Java programs that are already compiled which also creates dynamic web content.
- The second difference between Servlet vs JSP is that JSP is actually translated and compiled into Servlet by web container when the first request comes for it, on the other hand, Servlet is already a Java program, whose instance is created and managed by the web container.
- Since JSP is translated and compiled into Servlet, they run faster compared to JSP.
- JSP was designed for HTML developer who doesn't know Java but familiar with the tag-based markup language like HTML, while Servlet is designed for Java developers who like to code in Java.
In MVC design pattern, JSP (Java Server Pages) act as a view and servlet act as a Controller. - JSP are generally preferred when there is not much processing of data required. But Servlets are best for use when there are more processing and manipulation involved. Your JSP should be as dumb as possible i.e. it should not contain any logic, all logic should go to Servlets. The job of JSP should just to display the data provided via model to it. If your JSP starts containing logic then it would be difficult to maintain.
- The advantage of JSP programming over Servlet is that we can build custom tags which can directly call Java beans. There is no such facility in servlets. On the other JSTL is the popular tag library which allows you to completely remove Java from JSP. By using expression language and JSTL core tag library, you can make your JSP free of Java. If you want to learn more about JSTL core tag library I suggest you read Head First Servlet and JSP, it has explained core tags from JSTL very well.
- We can achieve the functionality of JSP at the client side by running JavaScript at client side. There are no such methods for servlets.
9. Difference between Constructor and init method in Servlet?
In real world application, you better use init()
method for initialization, because init()
method receives a ServletConfig parameter, which may contain any initialization parameters for that Servlet from web.xml file. Since web.xml provides useful information to web container e.g. name of Servlet to instantiate, ServletConfig instance is used to supply initialization parameter to Servlets. You can configure your Servlet based upon settings provided in ServletConfig object e.g. you can also provide environment specific settings e.g. path of temp directory, database connection parameters (by the way for that you should better leverage JNDI connection pool) and any other configuration parameters. You can simply deploy your web application with different settings in web.xml file on each environment. Remember, init()
method is not chained like constructor, where super class constructor is called before sub class constructor executes, also known as constructor chaining.
How to disable submit button using JavaScript?
You don't need to do a lot just add this.disabled='disabled'
on onclick event handler of button like below:
<form action="submit.jsp" method="post" > <input type="submit" name="SUBMIT" value="Submit Form" onclick="this.disabled='disabled'" /> </form>
This JavaScript code will disable submit button once clicked. Instead of this, which represent current element similar to Java this keyword, You can also use document.getElementById('id') but this is short and clear.
Now some browser has problem to submit data from disabled button. So, its better to call form.submit() from onclick itself to submit form data, which makes your code to onclick="this.disabled=true;this.form.submit(). Particularly, on Internet Explorer a disabled submit button doesn't submit form data to server. You can also change value or text of submit button from submit to "Submitting...." to indicate user that submit is in progress. Final JavaScript code should look like:
<form action="submit.jsp" method="post" > <input type="submit" name="SUBMIT" value="Submit Form" onclick="this.value='Submitting ..';this.disabled='disabled'; this.form.submit();" /> </form>
That's all you need to disable submit button using HTML and JavaScript to prevent multiple submission.
10. Difference between URL Rewriting and URL Encoding in JSP Servlet?
1) java.servlet.http.HttpServletResponse
methods encodeURL(String url) and encodeRedirectURL(String URL) is used to encode SesssionID on URL to support URL-rewriting. don't confuse with name encodeURL() because it doesn't do URL encoding instead it embeds sessionID in url if necessary. logic to include sessionID is in method itself and it doesn't embed sessionID if browser supports cookies or session maintenance is not required. In order to implement a robust session tracking all URL from Servlet and JSP should have session id embedded on it.
In order to implement URL-rewriting in JSP you can use use JSTL core tag all URL passed to it will automatically be URL-rewriting if browser doesn't support cookies.
While java.net.URLEncoder.encode()
and java.net.URLDecoder.decode()
is used to perform URL Encoding and decoding which replace special character from String to another character. This method uses default encoding of system and also deprecated instead of this you can use java.net.URLEncoder.encode(String URL, String encoding) which allows you to specify encoding. as per W3C UTF-8 encoding should be used to encode URL in web application.
2) In URL rewriting session id is appended to URL and in case of URL-encoding special character replaced by another character.
11. Tomcat – java.lang.OutOfMemoryError: PermGen space Cause and Solution?
Find the offending classes which are retaining reference of Classloader and preventing it from being garbage collected. Tomcat provides memory leak detection functionality after tomcat 6 onwards which can help you to find when the particular library, framework or class is causing a memory leak in tomcat. Here are some of the common causes of java.lang.OutOfMemoryError: PermGen space in tomcat server:
tomcat java.lang.outofmemoryerror:permgen space solution
1) JDBC Drivers:
JDBC drivers are most common cause of java.lang.OutOfMemoryError: PermGen space in tomcat if web app doesn't unregister during the stop. One hack to get around this problem is that JDBC driver to be loaded by common class loader than application classloader and you can do this by transferring driver's jar into tomcat lib instead of bundling it on web application's war file.
2) Logging framework:
A similar solution can be applied to prevent logging libraries like Log4j causing java.lang.OutOfMemoryError: PermGen space in tomcat.
3) Application Threads which have not stopped.
Check your code carefully if you are leaving your thread unattended and running in a while loop that can retain classloader's reference and cause java.lang.OutOfMemoryError: PermGen space in tomcat web server. Another common culprit is ThreadLocal, avoid using it until you need it absolutely, if do you make sure to set them null or free any object ThreadLocal variables are holding.
Another Simple Solution is to increase PermGen heap size in catalina.bat or Catalina.sh of tomcat server; this can give you some breathing space but eventually, this will also return in java.lang.OutOfMemoryError: PermGen space after some time.
12. How to loop over HashMap in JSP using JSTL?
File Upload Example in Java using Servlet?
13. How can we create deadlock condition on our servlet?
one simple way to call doPost()
method inside doGet()
and doGet()
method inside doPost()
it will create deadlock situation for a servlet.
Why super.init (config) is the first statement inside init(config) method?
This will be the first statement if we are overriding the init(config) method by this way we will store the config object for future reference and we can use by getServletConfig() to get information about config object if will not do this config object will be lost and we have only one way to get config object because servlet pass config object only in init method . Without doing this if we call the ServletConfig method will get NullPointerException.
14. Why we need to implement Single Thread model in the case of Servlet?
In J2EE we can implement our servlet in two different ways either by using:
- Single Thread Model
- Multithread Model
Depending upon our scenario, if we have implemented single thread means only one instance is going handle one request at a time no two thread will concurrently execute service method of the servlet.
The example in banking accounts where sensitive data is handled mostly this scenario was used this interface is deprecated in Servlet API version 2.4.
As the name signifies multi-thread means a servlet is capable of handling multiple requests at the same time. This servlet interview question was quite popular few years back on entry level but now it's losing its shine.
15. What is the difference between ServletConfig and ServletContext?
ServletConfig as the name implies provide the information about the configuration of a servlet which is defined inside the web.xml file or we can say deployment descriptor.its a specific object for each servlet.
ServletContext is an application specific object which is shared by all the servlet belongs to one application in one JVM .this is a single object which represents our application and all the servlet access application specific data using this object.servlet also use their method to communicate with the container.
16. What is the difference between HttpServlet and GenericServlet in Servlet API?
GenericServlet provides framework to create a Servlet for any protocol e.g. you can write Servlet to receive content from FTP, SMTP etc, while HttpServlet is built-in Servlet provided by Java for handling HTTP requests. See detailed answer for deep discussion.
17. What are JSTL tags in jsp?
JSTL Core Tags: JSTL Core tags provide support for iteration, conditional logic, catch exception, url, forward or redirect response etc. To use JSTL core tags, we should include it in the JSP page like below.
<%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c" %>
JSTL Formatting and Localisation Tags: JSTL Formatting tags are provided for formatting of Numbers, Dates and i18n support through locales and resource bundles. We can include these jstl tags in JSP with below syntax:
<%@ taglib uri="http://java.sun.com/jsp/jstl/fmt" prefix="fmt" %>
JSTL SQL Tags: JSTL SQL Tags provide support for interaction with relational databases such as Oracle, MySql etc. Using JSTL SQL tags we can run database queries, we include these JSTL tags in JSP with below syntax:
<%@ taglib uri="http://java.sun.com/jsp/jstl/sql" prefix="sql" %>
JSTL XML Tags: JSTL XML tags are used to work with XML documents such as parsing XML, transforming XML data and XPath expressions evaluation. Syntax to include JSTL XML tags in JSP page is:
<%@ taglib uri="http://java.sun.com/jsp/jstl/xml" prefix="x" %>JSTL Functions Tags: JSTL tags provide a number of functions that we can use to perform common operation, most of them are for String manipulation such as String Concatenation, Split String etc. Syntax to include JSTL functions in JSP page is:
<%@ taglib uri="http://java.sun.com/jsp/jstl/functions" prefix="fn" %>
18. Give some example of JSTL core tags?
<c:out>
Like <%= ... >, but for expressions.
<c:set >
Sets the result of an expression evaluation in a 'scope'
<c:remove >
Removes a scoped variable (from a particular scope, if specified).
<c:catch>
Catches any Throwable that occurs in its body and optionally exposes it.
<c:if>
Simple conditional tag which evalutes its body if the supplied condition is true.
<c:choose>
Simple conditional tag that establishes a context for mutually exclusive conditional operations, marked by <when> and <otherwise>.
<c:when>
Subtag of <choose> that includes its body if its condition evalutes to 'true'.
<c:otherwise >
Subtag of <choose> that follows the <when> tags and runs only if all of the prior conditions evaluated to 'false'.
<c:import>
Retrieves an absolute or relative URL and exposes its contents to either the page, a String in 'var', or a Reader in 'varReader'.
<c:forEach >
The basic iteration tag, accepting many different collection types and supporting subsetting and other functionality .
<c:forTokens>
Iterates over tokens, separated by the supplied delimeters.
<c:param>
Adds a parameter to a containing 'import' tag's URL.
<c:redirect >
Redirects to a new URL.
<c:url>
Creates a URL with optional query parameters
No comments:
Post a Comment